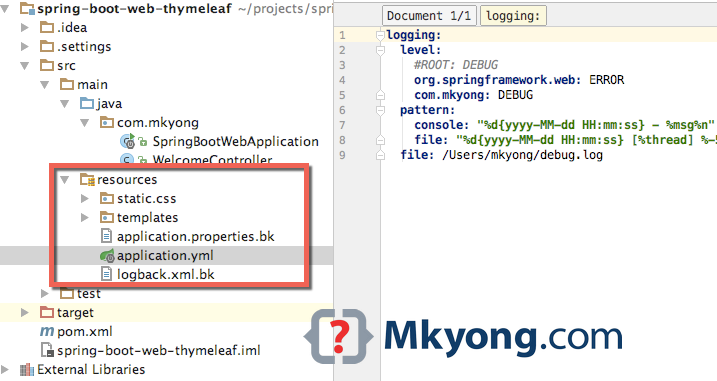
By default, the SLF4j Logging is included in the Spring Boot starter package.
spring-boot-web-project$ mvn dependency:tree
+...
+- org.springframework.boot:spring-boot-starter-logging:jar:1.4.2.RELEASE:compile
[INFO] | | | +- ch.qos.logback:logback-classic:jar:1.1.7:compile
[INFO] | | | | \- ch.qos.logback:logback-core:jar:1.1.7:compile
[INFO] | | | +- org.slf4j:jcl-over-slf4j:jar:1.7.21:compile
[INFO] | | | +- org.slf4j:jul-to-slf4j:jar:1.7.21:compile
[INFO] | | | \- org.slf4j:log4j-over-slf4j:jar:1.7.21:compile
+...
Review this Spring Boot Logback XML template to understand the default logging pattern and configuration.
1. application.properties
To enable logging, create a application.properties
file in the root of the resources
folder.
1.1 logging.level
– define logging level, the logging will be output to console.
logging.level.org.springframework.web=ERROR
logging.level.com.mkyong=DEBUG
1.2 logging.file
– define logging file, the logging will be output to a file and console.
logging.level.org.springframework.web=ERROR
logging.level.com.mkyong=DEBUG
#output to a temp_folder/file
logging.file=${java.io.tmpdir}/application.log
#output to a file
#logging.file=/Users/mkyong/application.log
1.3 logging.pattern
– define a custom logging pattern.
logging.level.org.springframework.web=ERROR
logging.level.com.mkyong=DEBUG
# Logging pattern for the console
logging.pattern.console= "%d{yyyy-MM-dd HH:mm:ss} - %msg%n"
# Logging pattern for file
logging.pattern.file= "%d{yyyy-MM-dd HH:mm:ss} [%thread] %-5level %logger{36} - %msg%n"
logging.file=/Users/mkyong/application.log
2. application.yml
This is the equivalent in YAML format.
logging:
level:
org.springframework.web: ERROR
com.mkyong: DEBUG
pattern:
console: "%d{yyyy-MM-dd HH:mm:ss} - %msg%n"
file: "%d{yyyy-MM-dd HH:mm:ss} [%thread] %-5level %logger{36} - %msg%n"
file: /Users/mkyong/application.log
3. Classic Logback.xml
If you don’t like the Spring Boot logging template, just create a standard logback.xml
in the root of the resources
folder or root of the classpath. This will override the Spring Boot logging template.
<configuration>
<property name="DEV_HOME" value="c:/logs" />
<appender name="FILE-AUDIT"
class="ch.qos.logback.core.rolling.RollingFileAppender">
<file>${DEV_HOME}/debug.logfile>
<encoder class="ch.qos.logback.classic.encoder.PatternLayoutEncoder">
<Pattern>
%d{yyyy-MM-dd HH:mm:ss} - %msg%n
Pattern>
encoder>
<rollingPolicy class="ch.qos.logback.core.rolling.TimeBasedRollingPolicy">
<fileNamePattern>${DEV_HOME}/archived/debug.%d{yyyy-MM-dd}.%i.log
fileNamePattern>
<timeBasedFileNamingAndTriggeringPolicy
class="ch.qos.logback.core.rolling.SizeAndTimeBasedFNATP">
<maxFileSize>10MBmaxFileSize>
timeBasedFileNamingAndTriggeringPolicy>
rollingPolicy>
appender>
<logger name="com.mkyong" level="debug"
additivity="false">
<appender-ref ref="FILE-AUDIT" />
logger>
<root level="error">
<appender-ref ref="FILE-AUDIT" />
root>
configuration>
4. Spring Boot logging by Profile
Read this article – Profile-specific configuration
Create a logback-spring.xml
in the root of the classpath, to take advantage of the templating features provided by Spring Boot.
In below example :
- If the profile is
dev
, logs to console and a rolling file. - If the profile is
prod
, logs to a rolling file only.
<configuration>
<include resource="org/springframework/boot/logging/logback/defaults.xml"/>
<property name="LOG_FILE" value="${LOG_FILE:-${LOG_PATH:-${LOG_TEMP:-${java.io.tmpdir:-/tmp}}/}spring.log}"/>
<springProfile name="dev">
<include resource="org/springframework/boot/logging/logback/console-appender.xml"/>
<appender name="ROLLING-FILE"
class="ch.qos.logback.core.rolling.RollingFileAppender">
<encoder>
<pattern>${FILE_LOG_PATTERN}pattern>
encoder>
<file>${LOG_FILE}file>
<rollingPolicy class="ch.qos.logback.core.rolling.TimeBasedRollingPolicy">
<fileNamePattern>${LOG_FILE}.%d{yyyy-MM-dd}.logfileNamePattern>
rollingPolicy>
appender>
<root level="ERROR">
<appender-ref ref="CONSOLE"/>
<appender-ref ref="ROLLING-FILE"/>
root>
springProfile>
<springProfile name="prod">
<appender name="ROLLING-FILE"
class="ch.qos.logback.core.rolling.RollingFileAppender">
<encoder>
<pattern>${FILE_LOG_PATTERN}pattern>
encoder>
<file>${LOG_FILE}file>
<rollingPolicy class="ch.qos.logback.core.rolling.TimeBasedRollingPolicy">
<fileNamePattern>${LOG_FILE}.%d{yyyy-MM-dd}.%i.gzfileNamePattern>
<timeBasedFileNamingAndTriggeringPolicy
class="ch.qos.logback.core.rolling.SizeAndTimeBasedFNATP">
<maxFileSize>10MBmaxFileSize>
timeBasedFileNamingAndTriggeringPolicy>
rollingPolicy>
appender>
<root level="ERROR">
<appender-ref ref="ROLLING-FILE"/>
root>
springProfile>
configuration>
spring:
profiles:
active: prod
logging:
level:
ROOT: ERROR
org.springframework: ERROR
org.springframework.data: ERROR
com.mkyong: INFO
org.mongodb: ERROR
file: /Users/mkyong/application.log
For non-web boot app, you can override the log file output like this :
$ java -Dlogging.file=/home/mkyong/app/logs/app.log -jar boot-app.jar
5. Set Root Level
# root logging level, warning : too much output
logging.level.=DEBUG
logging:
level:
ROOT: DEBUG